
Filtering Content The Easy Way
On a website such as this one, you sometimes want to dig further on a topic, such as Tailwind or Rails 7. With this in mind, website developers frequently apply tags to their content to make this easier. In this post, I will share a simple way to implement this!
Side note: On this website, I have implemented tags using the fantastic acts-as-taggable-on gem.
Since this site is focused on articles and blog posts, like the one you're reading now, the homepage defaults to the BlogPosts index page. When the user first hits the page, all published blog posts are displayed, usually with some code like the following:
# blog_posts_controller
Side note: On this website, I have implemented tags using the fantastic acts-as-taggable-on gem.
Since this site is focused on articles and blog posts, like the one you're reading now, the homepage defaults to the BlogPosts index page. When the user first hits the page, all published blog posts are displayed, usually with some code like the following:
# blog_posts_controller
def index @blog_posts = BlogPost.published end
Side note: The .published method is actually a custom scope, defined on the model, that allows me to choose the order in which the resulting blog posts will appear.
Users can view published blog posts, and that's all well and good, but they are viewing all blog posts. How do we allow them to filter the content in order to see something more relevant to their current interests? We can actually handle this in just a few steps.
First, we want the index action in our controller to watch for a parameter to appear in the URL. We'll call ours tag.
# blog_posts_controller
def index @blog_posts = BlogPost.published if params[:tag].present? @blog_posts = @blog_posts.tagged_with(params[:tag]) end end
Each tag that appears below an article, or in the list at the bottom of the index page, is a link that submits a get request to the index page and applies a tag parameter:
# _tags.html.erb
<%= link_to root_path(tag: tag.name) do %> <div class="your styles here"> <%= tag %> </div> <% end %>
Side note: Make sure you read this Stack Overflow post about SQL injection. You don't want to leave any open vector for hackers to manipulate your database.
When clicked, this redirects the user to http://website.com?tag=whatever and gets noticed by the controller, which then further filters the blog posts using the .tagged_with method provided to us by the aforementioned acts-as-taggable-on gem.
That's it!
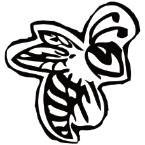